GraphQL is a declarative API query language that you can use to fetch data from a server. Facebook started its development in 2012 and open sourced it in 2015. Ever since a huge community and tooling ecosystem around GraphQL emerged.
Why GraphQL?
GraphQL is often considered to be a better alternative to REST, because…
- it gives you exactly the data you asked for (no overfetching of data) and thus reduces the required amount of data being sent over the wire
- You can avoid multiple REST calls. GraphQL contains all requested data in a single response.
- GraphQL does not need versioning because you can extend your API in a backwards compatible way
- Easy to learn and understand. Queries look very similar to the responses.
- You can wrap GraphQL around existing APIs to create a single API interface
- is language agnostic. You can use many languages to build a GraphQL server (yet in this article we will use JavaScript).
GraphQL vs Apollo
Apollo is a platform that uses GraphQL to allow you to build what they call a supergraph, which is like the big entry door to all your data, services and capabilities that you have. Apollo is not part of this series of articles but since it uses GraphQL all of the articles here are relevant for developing with Apollo too.
A quick overview on how GraphQL works
From your client application you send requests that are written in the specific GraphQL query language which uses queries to fetch data, mutations to create/update/delete data or subscriptions to observe data changes. More precisely, you request fields
from an object on the backend. Request are commonly sent using a GraphQL client library such as Apollo or Relay to a single /graphql
endpoint provided by your GraphQL server. That server is composed of a single Schema with many Types. A schema creates a strongly typed relationship between client and server. The GraphQL Execution Engine parses the client query and validates the schema, then each field that was sent to the server will get resolved to a scalar value using resolver functions. A resolver functions usually queries a database to “resolve to” a value, but it can be anything such as requests to other APIs. This “resolving” is done for each field in sequence and makes use of batched resolving, which effectively prevents the need to make additional requests for fields that have already been resolved. Finally, results are returned as a JSON response that conforms to the GraphQL schema.
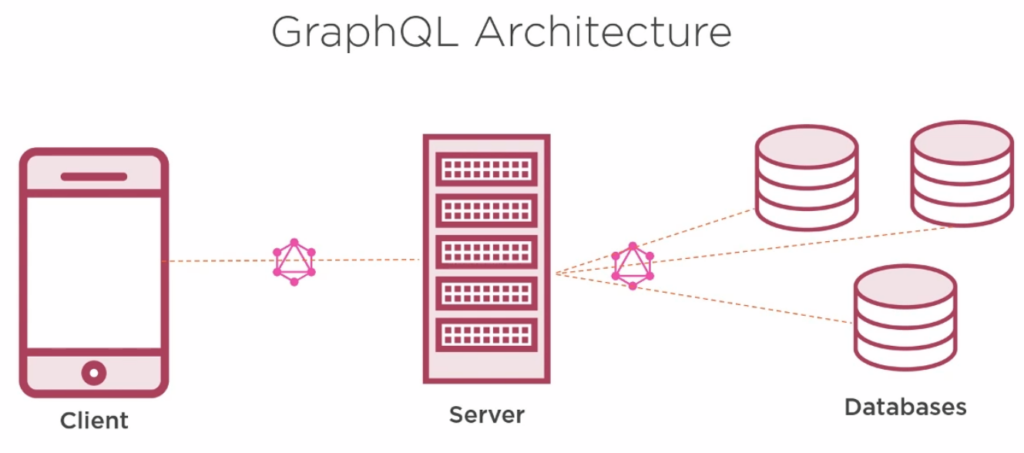
Client sends queries, mutations or subscriptions in one request:
query { book } mutation { addBook(title: "My book") } subscription PostFeed { postCreated { author comment } }
Server receives client request and matches it against its schema:
type Query { book: Book } type Mutation { addBook(title: String!) } type Subscription { postCreated: Post }
GraphQL Tools
- GraphiQL is an in-browser IDE for writing, live validating and testing GraphQL queries
- GraphQL Voyager to visualize the GraphQL API as an interactive graph
- GraphQL Visual Editor creates schemas by joing visual blocks together and then transforms them into code
- GraphQL Faker mocks your API with realistic data from faker.js and it is useful for testing and coding against future APIs