There are many strategies to scale your application:
- Cloning
- Decomposing
- Splitting
The cluster
module helps you to scale your code on one system that has many CPU cores. The idea is: You have one master process and clone your code by forking as many worker processes as you have CPU cores in your system. This will allow the master process to to distribute computation (e.g. incoming web requests) to the worker processes in a round-robin manner. Each worker process is completely independent, having its own event loop, memory and node environment.
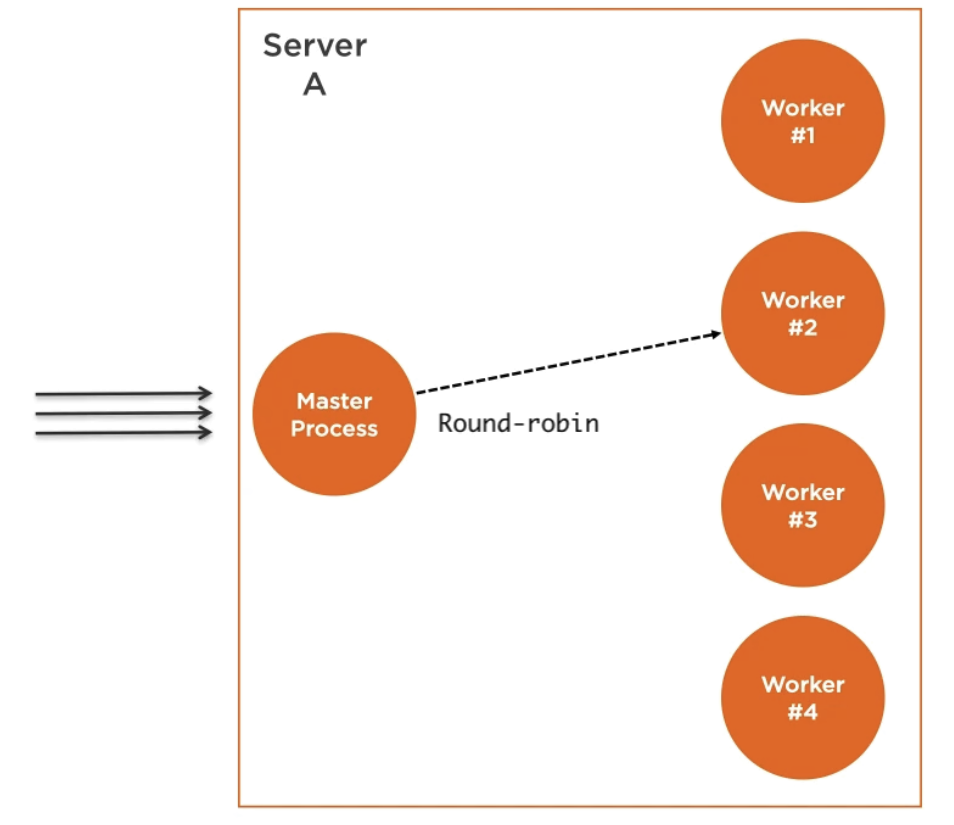
Even on ‘small’ machines with only a few CPU cores it makes sense to enable clustering to increase fault-tolerance and load balancing. To make it even easier you can use a process manager like pm2.
Load balancing example
Let’s assume you have a computational intensive HTTP process:
const http = require('http'); const pid = process.pid; const server = http.createServer((req, res) => { for (let i=0; i<1e7; i++); // simulate CPU work res.end(`Handled by process ${pid}`); }); server.listen(8000, () => { console.log(`Started process ${pid}`); })
It would output something like Handled by process 16324
on every browser refresh.
Now we want this to be load balanced to different worker processes, therefore we create a cluster:
const cluster = require('cluster'); const os = require('os'); if (cluster.isMaster) { const cpus = os.cpus().length; console.log(`Forking for ${cpus} CPUs`); for (let i = 0; i < cpus; i++) { cluster.fork(); } } else { require('./server'); }
You can access a list of all forked workers with cluster.workers
. So if you want to send a message to all workers (broadcasting) you simply loop over them:
Object.values(cluster.workers).forEach(worker => { work.send('Hello'); });