V8 and its feature groups
Node’s build-in JavaScript engine is V8, but others such as Chakra (by Microsoft) can be used. V8 has three feature groups:
- Shipping (stable features, enabled by default)
- Staged (almost stable, needs
--harmony
flag to be enabled) - in Progress (experimental, also needs specific flags to be enabled)
See all options via node --v8-options
. You can set those options when starting node or also at runtime using v8
module.
Executing code
Flow of code execution
The execution flow of your code works like this: Your code is interpreted by V8, which accesses Core Modules, C++ Bindings or libuv which provides the event loop and abstracts non-blocking IO operations. Those interact with the underlying operating system. Node itself has dependencies to openssl, zlip, ares, and others. You can get their versions with node -p "process.versions"
.
Running one-line code
You can run a one line code via node -p "process.arch"
. You can --require
(-r
) a module beforehand.
Using the REPL to run code
For a bit more extensive code you can start a REPL (Read-Eval-Print-Loop) by simply entering node
on a command line. Now simply start writing and invoking your JS code. This even works when starting a function on line 1 and continue to define it on line 2 and so on.
You exit the REPL with CTRL+D
or by entering .exit
. You simply clear the REPL with CTRL+L
. If you prefer to enter code multi-line (instead of line-by-line) you can enter .editor
. If you messed up what you wrote you can .break
out of it. More options are displayed with .help
. You can save everything you wrote in the REPL to a file with .save my.js
and .load my.js
any time later on again. You can use the convenient reverse search in the REPL by pressing CTRL+R
.
Press Tab twice to get a list of all possible commands. Press Tab once for autocompletion of whatever you already typed in.
The underscore _
stores the return value of the previous expression, for example:
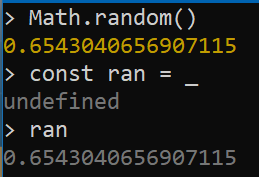
There is also a REPL module that lets you create customized REPL sessions, for example use streams instead of stdin/stdout or add other modules to the global context.
Clearing the console output
process.stdout.write('\u001B[2J\u001B[0;0f'); process.stdout.write('Test'); process.stdout.write('\n\> ');